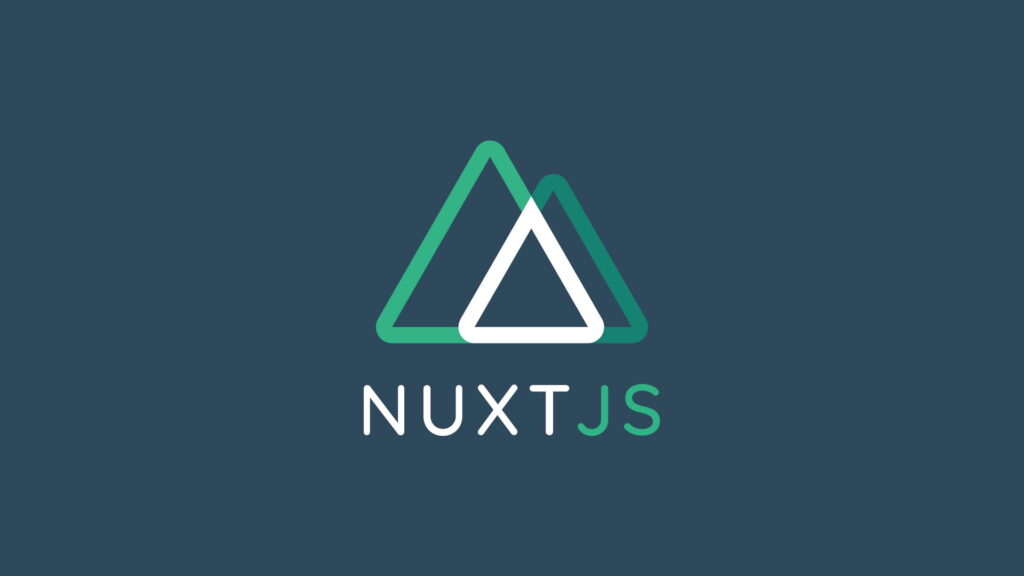
@PropSyncとは?
@PropSync
は、Vue.jsで使用されるデコレータの1つで、
親コンポーネントと子コンポーネントのプロパティを同期させるために使用されます。
子コンポーネントにプロパティを渡し、子コンポーネントでプロパティが変更された場合に、
その変更を親コンポーネントに反映することができます。
例えば、以下のような親コンポーネントと子コンポーネントがあるとします。
// 親コンポーネント
<template>
<div>
<h1>{{title}}</h1>
<child-component :count="count" @update:count="onCountUpdate" />
</div>
</template>
<script lang="ts">
import { Component, Vue } from 'vue-property-decorator';
import ChildComponent from './ChildComponent.vue';
@Component({
components: {
ChildComponent,
},
})
export default class ParentComponent extends Vue {
title = 'Parent Component';
count = 0;
onCountUpdate(count: number) {
this.count = count;
}
}
</script>
// 子コンポーネント
<template>
<div>
<button @click="incrementCount">{{count}}</button>
</div>
</template>
<script lang="ts">
import { Component, Prop, Vue } from 'vue-property-decorator';
@Component
export default class ChildComponent extends Vue {
@Prop(Number) count!: number;
incrementCount() {
this.$emit('update:count', this.count + 1);
}
}
</script>
子コンポーネントでカウントアップボタンをクリックすると、count
プロパティが1増加します。
この変更が、@PropSync
デコレータを使用して、親コンポーネントのcount
プロパティに同期されます。
次に、@PropSync
デコレータを使用して、子コンポーネントでcount
プロパティを同期する例です。
// 親コンポーネント
<template>
<div>
<h1>{{title}}</h1>
<child-component :count.sync="count" />
</div>
</template>
<script lang="ts">
import { Component, Vue } from 'vue-property-decorator';
import ChildComponent from './ChildComponent.vue';
@Component({
components: {
ChildComponent,
},
})
export default class ParentComponent extends Vue {
title = 'Parent Component';
count = 0;
}
</script>
// 子コンポーネント
<template>
<div>
<button @click="incrementCount">{{count}}</button>
</div>
</template>
<script lang="ts">
import { Component, Prop, Vue } from 'vue-property-decorator';
@Component
export default class ChildComponent extends Vue {
@PropSync('count', { type: Number })
syncedCount!: number;
javascriptCopy codeincrementCount() {
this.syncedCount += 1;
}
}
</script>
この例では、@PropSync
デコレータがcount
プロパティを同期させるために使用されています。
子コンポーネントのsyncedCount
プロパティを増加させることで、親コンポーネントのcount
プロパティが同期されます。
.sync装飾子とは?
.sync
は、Vue.jsのシンタックスシュガーで、@PropSync
デコレータと同様の機能を提供します。
.sync
は、子コンポーネントから親コンポーネントにプロパティを更新するために使用されます。
以下は、.sync
シンタックスを使用して、子コンポーネントから親コンポーネントにプロパティを更新する例です。
// 親コンポーネント
<template>
<div>
<h1>{{title}}</h1>
<child-component :count.sync="count" />
</div>
</template>
<script lang="ts">
import { Component, Vue } from 'vue-property-decorator';
import ChildComponent from './ChildComponent.vue';
@Component({
components: {
ChildComponent,
},
})
export default class ParentComponent extends Vue {
title = 'Parent Component';
count = 0;
}
</script>
// 子コンポーネント
<template>
<div>
<button @click="$emit('update:count', count + 1)">{{count}}</button>
</div>
</template>
<script lang="ts">
import { Component, Prop, Vue } from 'vue-property-decorator';
@Component
export default class ChildComponent extends Vue {
@Prop(Number) count!: number;
}
</script>
この例では、.sync
シンタックスを使用して、子コンポーネントでcount
プロパティを同期させています。
子コンポーネントのカウントアップボタンがクリックされると、
.sync
シンタックスによって親コンポーネントのcount
プロパティが更新されます。
まとめ
@PropSync
デコレータと.sync
シンタックスは、
Vue.jsで親コンポーネントと子コンポーネントのプロパティを同期させるために使用されます。
.sync
シンタックスは、@PropSync
デコレータと同様の機能を提供しますが、シンプルな構文を提供します。
どちらの方法でも、Vue.jsでプロパティを同期することができます。